How to send emails in .NET Core with MimeKit and Mailkit
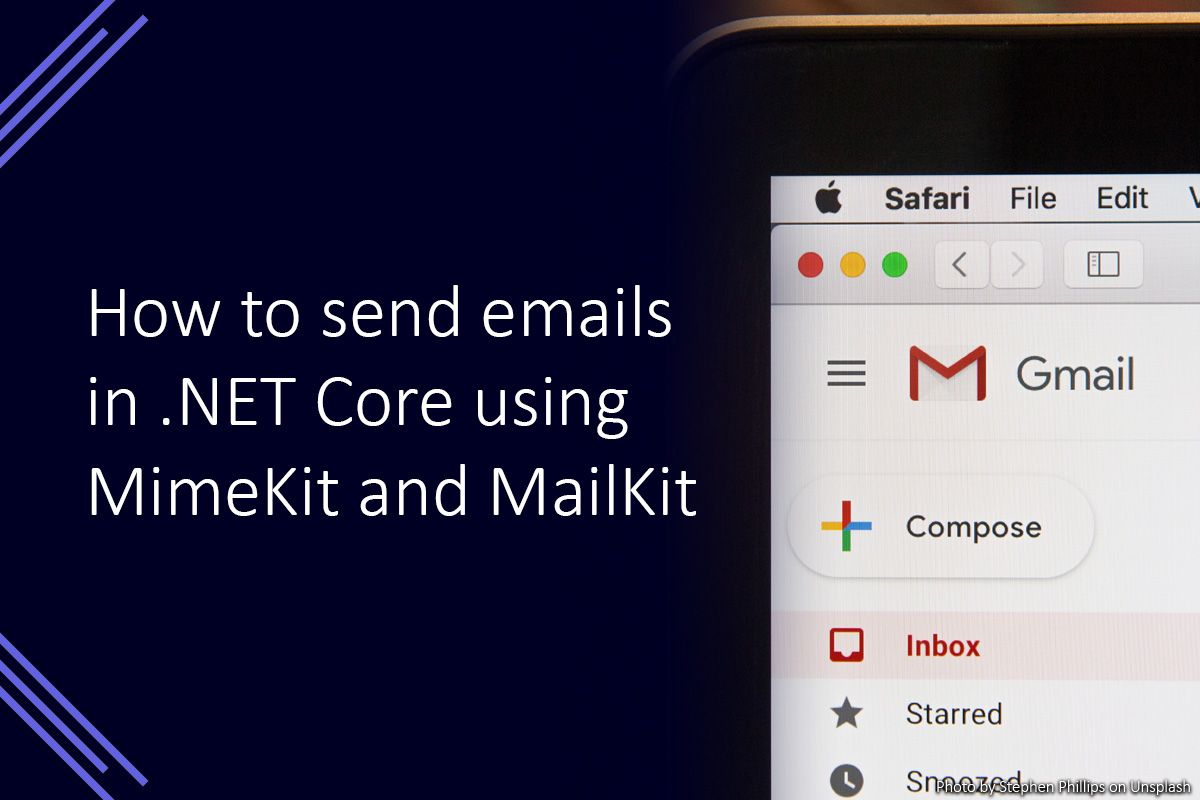
The ability for an application to send emails is a very common requirement. This can be for emails such as providing account information, sending marketing emails or even a password reset email.
This post will cover how to send an email using MimeKit and MailKit from an ASP.NET Core MVC web application.
I will use MailSlurper as a local SMTP server to test the sending of the emails. You can use any SMTP server you prefer though.
Creating the ASP.NET Core MVC demo project
I am going to create a demo MVC project named ‘MimeKitDemo’ for this post. You can use any project you would like.
You can create a project through Visual Studio or by using the following command on the .NET CLI.
dotnet new mvc –name MimeKitDemo
Installing MimeKit and MailKit
The first step is to add the MimeKit and MailKit packages to the project. This can be done through the Visual Studio Package Manager or the .NET CLI.
dotnet add package MimeKit dotnet add package MailKit
Setting up an email
I will use the Index action in the HomeController
for this example. You can put the code wherever is appropriate for your application.
The first step is to create a new MimeMessage
for the email we will send. Then we can set from address, recipients and email subject.
var message = new MimeMessage();
message.From.Add(new MailboxAddress("Test From Address", "noreply@test.com"));
message.To.Add(new MailboxAddress("Test Recipient", "recipient@test.com"));
message.Subject = "Test Email Subject";
Now the email has been setup we can create the body (the actual message).
Creating the email body
MimeKit includes a BodyBuilder
class to help with creating the body of an email. This class allows you to easily create a HTML and plain text equivalent of your message.
var bodyBuilder = new BodyBuilder();
bodyBuilder.TextBody = @"
Hi!
This is just a test email so feel free to ignore it!
Thanks! :)";
bodyBuilder.HtmlBody = @"
<html>
<body>
<h1>Test Email</h1>
<p>Hi!</p>
<p>This is just a <strong>test email</strong> so feel free to ignore it!</p>
<p>Thanks! :)</p>
</body>
</html>";
message.Body = bodyBuilder.ToMessageBody();
Now the email is ready, it is time to send it.
Sending the email
To do this we need to connect to the SMTP server, send the email to the server and then disconnect.
using (var smtpClient = new SmtpClient())
{
await smtpClient.ConnectAsync("localhost", 2500);
await smtpClient.SendAsync(message);
await smtpClient.DisconnectAsync(true);
}
After that you should receive the email – hurray!
MimeMessage has many features which you can use for attaching images or files to emails.